Latest version
Game python raspberry-pi arduino sprites pygame animation-effects slot-machine dispenser halloween-theme coins-detector Updated May 30, 2020 Python. I'm programming a slot machine for school and cannot get the machine to re-run once it is finished. I am relatively new and would like some honest feedback. How can I get my program to re-run? This is the code I'm trying to do this with. I've just modified my code to look like this.
Released:
A multi-armed bandit library for Python
Project description
A multi-armed bandit library for Python
Slots is intended to be a basic, very easy-to-use multi-armed bandit library for Python.
Author
Roy Keyes -- roy.coding@gmail
License: MIT
See LICENSE.txt
Introduction
slots is a Python library designed to allow the user to explore and use simple multi-armed bandit (MAB) strategies. The basic concept behind the multi-armed bandit problem is that you are faced with n choices (e.g. slot machines, medicines, or UI/UX designs), each of which results in a 'win' with some unknown probability. Multi-armed bandit strategies are designed to let you quickly determine which choice will yield the highest result over time, while reducing the number of tests (or arm pulls) needed to make this determination. Typically, MAB strategies attempt to strike a balance between 'exploration', testing different arms in order to find the best, and 'exploitation', using the best known choice. There are many variation of this problem, see here for more background.
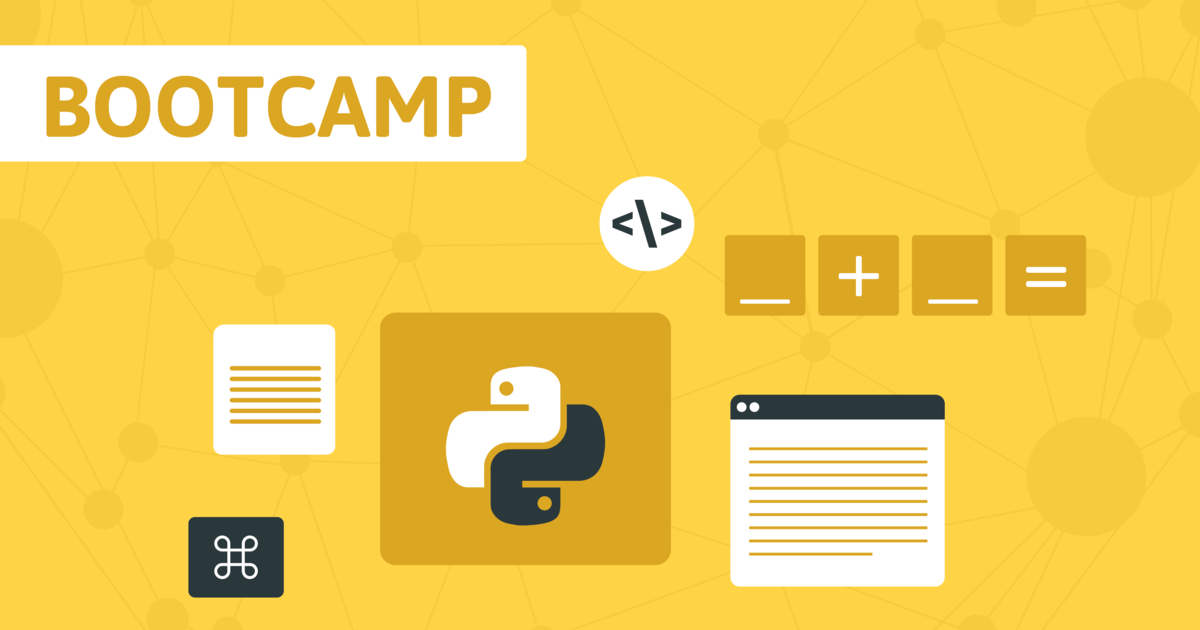
https://omgsiam.netlify.app/bovada-slot-machine-wins.html. slots provides a hopefully simple API to allow you to explore, test, and use these strategies. Basic usage looks like this:
Using slots to determine the best of 3 variations on a live website.
Make the first choice randomly, record responses, and input reward 2 was chosen. https://omgsiam.netlify.app/pompeii-slot-machine-for-iphone.html. Run online trial (input most recent result) until test criteria is met.
The response of mab.online_trial()
is a dict of the form:
Our platform is cryptographically signed to guarantee that what you download came directly from us and has not been corrupted or tampered with.All of your spin data is transmitted using the latest secure technology and is protected with the highest level SSL certificates.Your data is held in a secure database and your personal details are encrypted to ensure total peace of mind.Login to your account with your secure credentials from any computer so you can keep tracking all of your spins wherever you are. Security Is Our Highest PriorityWe have been dedicated in making sure our software is completely secure. Counting cash slot machine.
Where:
- If the criterion is met,
new_trial
=False
. choice
is the current choice of arm to try.best
is the current best estimate of the highest payout arm.
To test strategies on arms with pre-set probabilities:
To inspect the results and compare the estimated win probabilities versus the true win probabilities:
By default, slots uses the epsilon greedy strategy. Besides epsilon greedy, the softmax, upper confidence bound (UCB1), and Bayesian bandit strategies are also implemented.
Regret analysis
A common metric used to evaluate the relative success of a MAB strategy is 'regret'. This reflects that fraction of payouts (wins) that have been lost by using the sequence of pulls versus the currently best known arm. The current regret value can be calculated by calling the mab.regret()
method.
For example, the regret curves for several different MAB strategies can be generated as follows:
API documentation
For documentation on the slots API, see slots-docs.md.
Todo list
- More MAB strategies
- Argument to save regret values after each trial in an array.
- TESTS!
Contributing
I welcome contributions, though the pace of development is highly variable. Please file issues and submit pull requests as makes sense.
Wolf Run is a 5-reel, 40-payline slot game developed by IGT.This game is centered around wolves. Going by today's standards, the graphics appear to be a little bit dated, but the gameplay is what will get you. Wolf Run is a 40 pay-line slot machine game produced by IGT. The game is based around a mystical, native american theme, featuring wolves, Indian dream catchers and the full moon as the major winning symbols. The main features of the Wolf Run slot are the stacked wilds and free. Like other online casino games, the wolf run casino game is available on Windows and Mac as well as on mobile at Ignition online casino website. If you decide to venture into the game, you can play wolf run slot for free. With the free wolf run slot machine. Free wolf run casino slots. Play Wolf Run Slot for Free. Try the online casino game totally free, No download, No Registration and No Deposit needed. Wolf Run Slot ️ Free Play Online Casino Game IGT Casino Robots ⚡ Play now Huge types of free casino games Free slots.
The current development environment uses:
- pytest >= 5.3 (5.3.2)
- black >= 19.1 (19.10b0)
- mypy = 0.761
You can pip install these easily by including dev-requirements.txt
.
For mypy config, see mypy.ini
. For black config, see pyproject.toml
.
Release historyRelease notifications | RSS feed
0.4.0
0.3.1
0.3.0
0.2.0
0.1.0
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size slots-0.4.0-py3-none-any.whl (8.7 kB) | File type Wheel | Python version py3 | Upload date | Hashes |
Filename, size slots-0.4.0.tar.gz (174.8 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for slots-0.4.0-py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | 2bc3e51a6223ae3984476f5d1bb272e70ca6d74fbd298fe48c7283cc1c358cc7 |
MD5 | 1ec6d4398f2a36036f452a9c77d0d43c |
BLAKE2-256 | 61dacfca624262fdcce7c043b5e96f06a64019d4a6581a31d5e34ee52b9d30cd |
Hashes for slots-0.4.0.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | b02b7b60084b6fe2b0297a14493289c4f7ee88ae374ec518237ca6b13d39e7ae |
MD5 | f91161c95cf32357b2b23b01ca4041c9 |
BLAKE2-256 | 443e20fea85cf3b7054cd90da868b50640e9dc532509567462e623ea1aeefb42 |
Slot Machine Programming Code Python Compiler
Slot machines are the most popular game in modern casinos. If you’ve never seen one, a slot machine resembles an arcade game that has a lever on its side. For a small fee you can pull the lever, and the machine will generate a random combination of three symbols. If the correct combination appears, you can win a prize, maybe even the jackpot.
Slot machines make fantastic profits for casinos because they offer a very low payout rate. In many games, such as Blackjack and Roulette, the odds are only slightly stacked in the casino’s favor. In the long run, the casino pays back 97 to 98 cents in prizes of every dollar that a gambler spends on these games. With slot machines, it is typical for a casino to only pay back 90 to 95 cents—and the casino keeps the rest. If this seems underhanded, keep in mind that slot machines are one of the most popular games at a casino; few people seem to mind. And if you consider that state lotteries have payout rates that are much closer to 50 cents on the dollar, slot machines don’t look that bad.
In this project, you will build a real, working slot machine modeled after some real life Video Lottery Terminals from Manitoba, Canada. The terminals were a source of scandal in the 1990s. You’ll get to the bottom of this scandal by writing a program that recreates the slot machines. You’ll then do some calculations and run some simulations that reveal the true payout rate of the machines.
This project will teach you how to write programs and run simulations in R. You will also learn how to:
Slot Machine Programming Code Python Ide
- Use a practical strategy to design programs
- Use
if
andelse
statements to tell R what to do when - Create lookup tables to find values
- Use
for
,while
, andrepeat
loops to automate repetitive operations - Use S3 methods, R’s version of Object-Oriented Programming
- Measure the speed of R code
- Write fast, vectorized R code